Using fonts
Canva has an expansive library of fonts. Apps can access and use a limited subset of these fonts when creating or updating text in a user's design.
Our design guidelines help you create a high-quality app that easily passes app review.
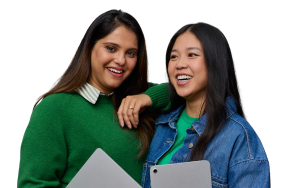
Open font picker
Apps can open a font picker that lets users choose a font from Canva's library. This offers a familiar and consistent user experience, and it's how we recommend most apps implement a font selection interface.
import { Button, Rows, Text } from "@canva/app-ui-kit";import { requestFontSelection } from "@canva/asset";import * as React from "react";import * as styles from "styles/components.css";export function App() {async function handleClick() {const fontResponse = await requestFontSelection();console.log(fontResponse);}return (<div className={styles.scrollContainer}><Rows spacing="1u"><Button variant="primary" onClick={requestFontSelection}>Open font picker</Button></Rows></div>);}
Remember font selection
When a user selects a font, we recommend storing that font in a state object. This has a few benefits:
- You can update the UI to display the selected font.
- You can access the selected font at other points in the lifecycle (e.g. when creating a text element).
- You can pass the selected font into the font picker, so the user's selection is remembered.
For example:
import { Button, Rows, Text } from "@canva/app-ui-kit";import { Font, requestFontSelection } from "@canva/asset";import * as React from "react";import * as styles from "styles/components.css";export function App() {// Keep track of the currently selected fontconst [selectedFont, setSelectedFont] = React.useState<Font | undefined>();const message = selectedFont? `The selected font is ${selectedFont.name}.`: `There is no font selected.`;async function handleClick() {const fontResponse = await requestFontSelection({selectedFontRef: selectedFont?.ref, // Specify the selected font, if one is defined});if (fontResponse.type !== "completed") {return;}// Update the currently selected fontsetSelectedFont(fontResponse.font);}return (<div className={styles.scrollContainer}><Rows spacing="1u"><Text>{message}</Text><Button variant="primary" onClick={handleClick}>Select a font</Button></Rows></div>);}
Use selected font
When a user selects a font, the app receives an asset reference for that font. To use the font, this reference can be passed into methods that accept a fontRef
property.
import { addElementAtPoint } from "@canva/design";await addElementAtPoint({type: "text",children: ["Hello world"],fontRef: selectedFont?.ref,});
Render font preview
All fonts have an associate image that contains a preview of the font. Apps can render this image to help users identify the currently selected font or to create a custom font picker.
<div>{selectedFont && <img src={selectedFont.previewUrl} />}</div>
Get list of recommended fonts
If the built-in font picker is not suitable for whatever reason, an app can alternatively:
- Request a list of recommended fonts from Canva.
- Create a custom user interface that renders those fonts.
The recommendations themselves are based on various factors, such as the user's locale.
import { findFonts } from "@canva/asset";const { fonts } = await findFonts();console.log(fonts); // => [ { name: "Arial", ... }]
We only recommend this option if it's strictly required for the app's use-case.
Get specific font details
An app can use a font's asset reference to get additional information about that font. Just keep in mind that asset references are short-lived and should only be used soon after the app receives them.
import { findFonts } from "@canva/asset";const { fonts } = await findFonts({ fontRefs: ["font_ref_goes_here"] });console.log(fonts); // => [ { name: "Arial", ... }]
Gotchas
- Apps can't access Canva Pro(opens in a new tab or window) fonts.
- Apps can't upload or otherwise define custom fonts.
- Apps can't download the underlying font files.
- Apps can only set the font of paragraphs, not of inline text.